フロントエンジニアになるための練習問題 押すと色が変わるボタン2
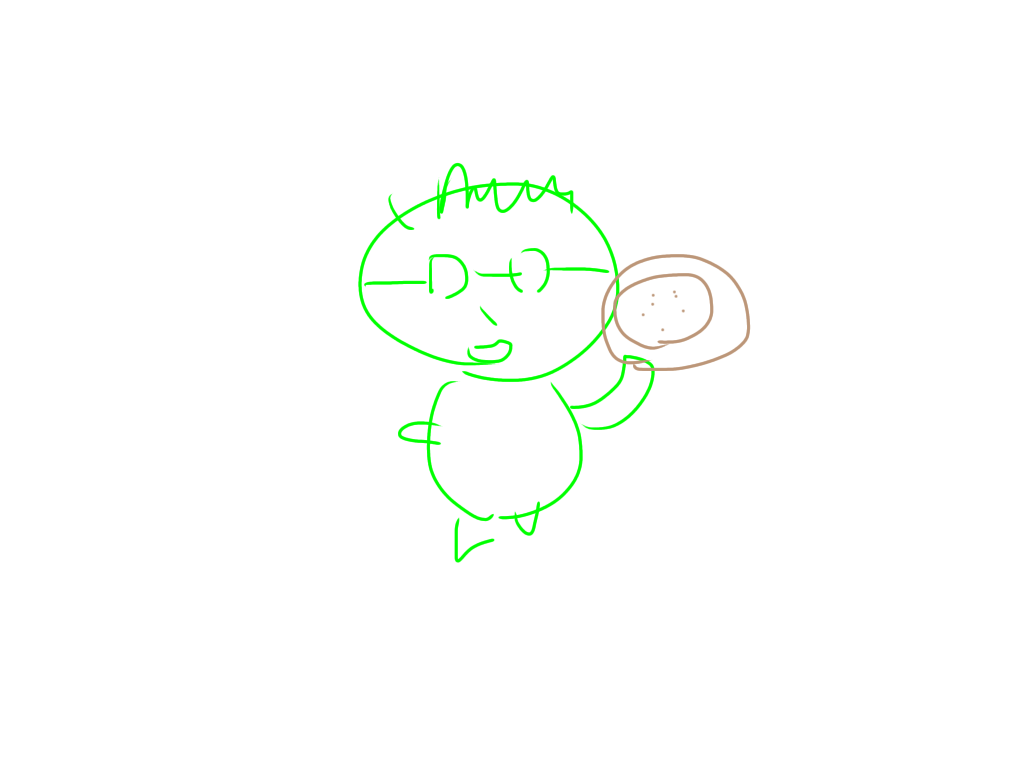
前回の課題の続編です。
課題
前回、ボタンを押すborderとテキストをredに変更するようにしましたが、もっとカラフルにして欲しいとの依頼がありました。
詳細は以下です。
- ボタンを押すと、赤、青、黄のいずれかの色に変わる
- どれに変わるかは完全にランダムであり、確率も一緒である
- もう一度ボタンを押すと、元の色(黒)に戻る
- さらにもう一度押すと、再び赤、青、黄のいずれかの色に変わる
前提・時間
- javascriptで条件分岐をかける
- 自分で調べて方法を見つけることができる
1時間程度。
実装の流れ
まずは、赤、青、黄のそれぞれのclassを定義します。
それから、ランダムな値を取得する関数を作ります。この関数は1から3のどれかをランダムに返す、というようにしてみます。
この関数をつかって1から3の値が得られたら、値をもとにどのclassを適用するかを条件分岐する処理を書きます。
あとはこれらの処理をボタンを押したときに実行するようにします。
最後に、赤、青、黄のいずれかのclassが適用されている場合は、すべてremoveする、という処理を書きます。
実装例
まずは、各classを定義してみます。
.my-btn {
width: 50px;
border: solid 2px lightslategray;
text-align: center;
}
/*追加*/
.red-active {
color: red;
border-color: red;
}
.blue-active {
color: blue;
border-color: blue;
}
.yellow-active {
color: yellow;
border-color: yellow;
}
続いて、ランダムな整数を返す関数を作成します。Googleで検索すると情報がでてきます。
const getRandom = (min, max) => Math.floor(Math.random() * (max - min + 1) + min);
続いて、値をもとにclassを返す関数を作成します。1が赤、2が青、3が黄のclass名を返すようにしています。
const getClass = (value) => {
switch (value) {
case 1: {
return 'red-active';
}
case 2: {
return 'blue-active';
}
case 3: {
return 'yellow-active';
}
}
};
あとは、ボタンを押した時の処理にこれらを書きます。全体のコードは以下です。
const getRandom = (min, max) => Math.floor(Math.random() * (max - min + 1) + min);
const getClass = (value) => {
switch (value) {
case 1: {
return 'red-active';
}
case 2: {
return 'blue-active';
}
case 3: {
return 'yellow-active';
}
}
};
const startButtonEl = document.getElementById("start-button");
startButtonEl.addEventListener("click", () => {
if(startButtonEl.classList.contains("red-active") || startButtonEl.classList.contains("blue-active") || startButtonEl.classList.contains("yellow-active")){
startButtonEl.classList.remove("red-active", "blue-active", "yellow-active");
}else{
const value = getRandom(1, 3);
const applyClass = getClass(value);
startButtonEl.classList.add(applyClass);
}
});
最近のコメント